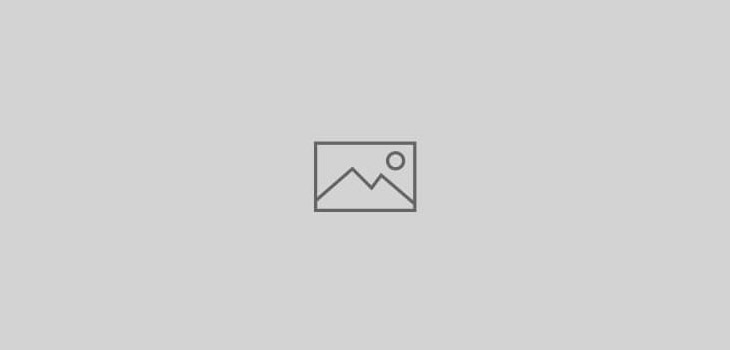
Using GeoIP database to identify site visitor’s country/city
GeoIP by Maxmind is offered in two versions – a paid and a free one. Currently GoeIP API ver. 2 is used to use either of those databases.
Maxmind’s URL for this is
http://dev.maxmind.com/geoip/geoip2/geolite2/
For this install we are using the free version following a guide found on these two pages:
http://ctrtard.com/code/how-to-install-the-maxmind-geoip2-database-for-php/
https://github.com/maxmind/GeoIP2-php
Before starting, we need to install curl and php-development tools
apt-get install curl php5-dev
I installed it on a Debian system and carried out all the install in /var/www folder.
I use the Country database. You can use the City one if you want/need
Here are the commands
cd /var/www/
sudo mkdir geoip2lite
cd geoip2lite/
sudo wget http://geolite.maxmind.com/download/geoip/database/GeoLite2-Country.mmdb.gz
sudo gunzip GeoLite2-Country.mmdb.gz
sudo mkdir /usr/local/share/GeoIP
sudo mv GeoLite2-Country.mmdb /usr/local/share/GeoIP/
This downloads the database from maxmind site, unpacks it and copies it to the folder from where it can be used
Next is to get the PHP API to use this database
sudo curl -sS https://getcomposer.org/installer | php
sudo php composer.phar require geoip2/geoip2:~2.0
Now cat or vim the composer.json file to make sure it has following lines. If it doesn’t, then add them manually
{
"require": {
"geoip2/geoip2": "~2.0"
}
}
and then
sudo php composer.phar install
By this time you should have following files and folders in your /var/www/geoip2lite folder
composer.json composer.lock composer.phar libmaxminddb vendor
Installing C libaray for faster lookups
cd libmaxminddb
sudo apt-get install git
sudo git clone --recursive https://github.com/maxmind/libmaxminddb
sudo apt-get install autoconf
sudo apt-get install dh-autoreconf
sudo ./bootstrap
sudo ./configure
sudo make check
sudo make install
sudo ldconfig
Installing PHP C Extension
cd ..
cd vendor
cd maxmind-db/reader/
cd ext
sudo phpize
sudo ./configure
sudo make
sudo make test
sudo make install
Now you got to add this extension in php.ini and to know where is the file located create a small phpinfo.php file with code below
<?php
// Show all information, defaults to INFO_ALL
phpinfo();
?>
load this file in browser and look for
Loaded Configuration File |
/etc/php5/fpm/php.ini |
Edit this file and add the following line at the end of file.
extension=maxminddb.so
And run this command
sudo ldconfig /usr/local/lib
Once done, reload apache by
sudo service apache2 restart
And check the phpinfo.php file again. You should search and find the following block
geoip
geoip support |
enabled |
geoip extension version |
1.1.0 |
geoip library version |
1006002 |
Directive |
Local Value |
Master Value |
geoip.custom_directory |
no value |
no value |
And it’s done
Now whichever folder (site) requires this GeoIP lookup, copy all composer files (composer.*) and vendor folder to that site’s folder.
You can test with following code in a test.php file
Remember to change the name of database file if you used the city database
<?php
require_once ‘vendor/autoload.php’;
use GeoIp2\Database\Reader;
$reader = new Reader(‘/usr/local/share/GeoIP/GeoLite2-Country.mmdb’);
$record = $reader->country($_SERVER[‘REMOTE_ADDR’]);
print($record->country->isoCode . “\n”);
print($record->country->name . “\n”);
?>